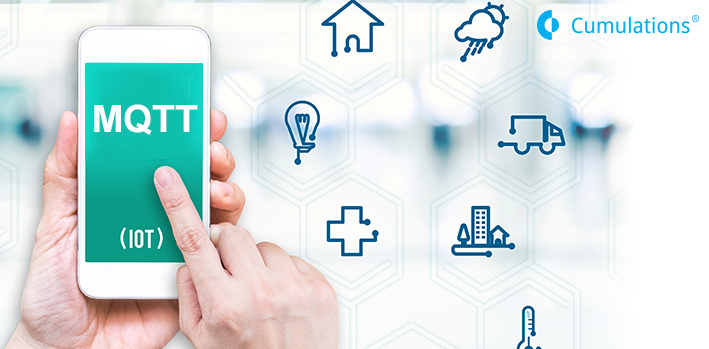
In the day of Internet of things, the most basic need is to get the sensors connected to the internet so that we get the required data from the sensors and also give our applications the ability to send instructions.
MQTT, though old technology, has found a market fit in IoT days due to its lower power usage, handling unreliable internet sources and also the advancements in cloud/mobile technologies. I would like to share a few pointers on the ways you could get your sensors internet enabled and make them talk to your applications.
1. Setting up an MQTT Broker
This is the important decision which would define the architecture of your product or solution. One can set up MQTT brokers in different ways.
- You can set up your own MQTT broker on your cloud or data centers. There is the wide range of open source brokers available like eMQTT, Mosquito etc.
- Cloud providers like Azure, AWS and IBM have their own IoT cloud infrastructure for developers to build their applications without worrying about the underlying platform. Also, these platforms have higher reliability and uptime guarantee. IBM Watson IoT platform is one such service.
2. Setting up publishers and subscribers
The publisher can be a sensor which would want to communicate its current state to all the subscribers subscribed to that topic (ex – current temperature from a sensor) or it can be an application which would want to change the state of a sensor from its current state (ex- close an opened window).
- Any gateway with a WiFi module can act as MQTT publisher or subscriber. There is the wide range of MQTT libraries available.
- Mobile applications can also act as publishers and subscribers using third party MQTT clients like MQTT-Client-Framework using the below steps. We have the code snippet to connect to IBM Watson IoT cloud from an iOS app.
//Authentication
MQTTSession *session = [[MQTTSession alloc] init];
session.delegate = self;
session.clientId = @“a:orgid:clientid"; //This id has to be unique.
session.userName = @“username";
session.password = @“password";
[session connectToHost:@“ORGID.messaging.internetofthings.ibmcloud.com" port:1883 usingSSL:NO];
[session connectWithConnectHandler:^(NSError *error) {
}];
//Subscribing to a topic
[session subscribeToTopic:@"iot-2/type/" atLevel:2 subscribeHandler:^(NSError *error, NSArray *gQoss){
if (error) {
NSLog(@"Subscription failed %@", error.localizedDescription);
} else {
NSLog(@"Subscription sucessfull! Granted Qos: %@", gQoss);
}
}];
Please note subscription should be done only after the connection is successful.
//After successfully subscribing to a topic, messages would be sent to the delegate method implemented which will have the message and topic details.
- (void)newMessage:(MQTTSession *)session data:(NSData *)data onTopic:(NSString *)topic qos:(MQTTQosLevel)qos
retained:(BOOL)retained mid:(unsigned int)mid {
// this is one of the delegate callbacks
NSString* newStr = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];
NSLog(@"topic is :%@ and got %@",topic,newStr);
}
We can also publish from an iOS app.
[_session publishData:postData onTopic:[NSString stringWithFormat:@"iot-2/type/Wi-Fimaster/id/%@/cmd/action/fmt/json",deviceId] retain:NO qos:MQTTQosLevelAtMostOnce publishHandler:^(NSError *error) {
if (error) {
NSLog(@"Publishing failed %@", error.localizedDescription);
} else {
NSLog(@"publishing sucessfull!");
}
}];
3. Subscribing from server application
Subscribing from mobile apps is not good enough. To update the current state of the sensors, it is important to subscribe from server applications because mobile apps will be suspended by the OS and subscription wouldn’t work when it is suspended. Hence to maintain the current state of all the sensors and at the right time, subscription from server application is a must. There are MQTT libraries available for different server side languages.