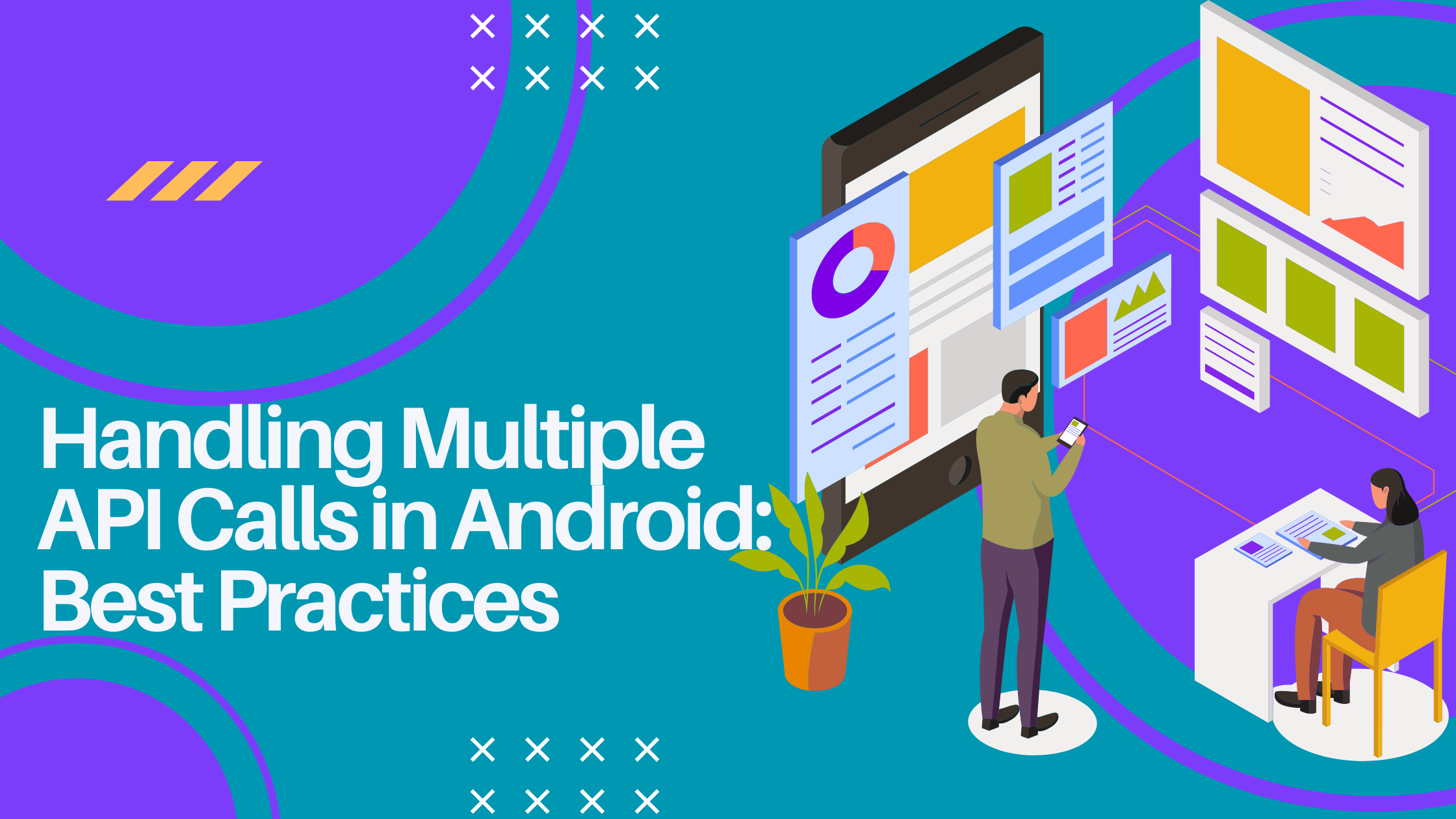
Handling multiple API calls in Android applications can sometimes be a challenging task due to the asynchronous nature of APIs. However, with the right approach, it can be managed effectively. In this article, we will explore different scenarios for handling multiple API calls and discuss best practices to ensure smooth execution and optimal performance.
Scenario 1: Sequential API Calls with Dependency
In certain cases, we may have API call B dependent on the result of API call A. To handle this scenario, we can utilize the power of async and await functionality. Consider the following code snippet:
fun getResultAResultB() { viewModelScope.launch { _genres.postValue(DataState.Init) _movieTitle.postValue(DataState.Init) val resultA = async { repository.getGenres() } _genres.postValue(resultA.await().convertToDataState()) val resultB = async { repository.getMovieTitles() } _movieTitle.postValue(resultB.await().convertToDataState()) } }
In the above code, we use coroutines to perform the API calls asynchronously. The async function is used to initiate each API call, and the await function suspends the coroutine until the respective API call is complete. This ensures that the second API call (B) is executed only after the first API call (A) has finished.
Scenario 2: Concurrent API Calls with Result Processing
In some scenarios, we need to execute multiple API calls concurrently and process the results together. The awaitAll function can be used to achieve this efficiently. Consider the following code snippet:
fun getGetResultAB() { viewModelScope.launch { _genres.postValue(DataState.Init) _movieTitle.postValue(DataState.Init) val resultA = async { repository.getGenres() } val resultB = async { repository.getMovieTitles() } val result = awaitAll(resultA, resultB) _genres.postValue(result[0].convertToDataState() as DataState<Genres>) _movieTitle.postValue(result[1].convertToDataState() as DataState<MovieTitles>) } }
In the above code, both API calls (A and B) are executed concurrently using coroutines and the awaitAll function. This allows us to await the completion of both API calls without any dependency. Once both results are available, they can be processed and assigned to their respective LiveData objects.
Scenario 3: Independent API Calls in Android for Performance
In certain situations, we may need to execute API calls independently and obtain results as soon as possible, without any strict ordering or dependency. Consider the following code snippet:
fun getPageAorPageB() { viewModelScope.launch { _genres.postValue(DataState.Init) repository.getGenres().let { result -> _genres.postValue(result.convertToDataState()) } }
viewModelScope.launch { _movieTitle.postValue(DataState.Init) repository.getMovieTitles().let { result -> _movieTitle.postValue(result.convertToDataState()) } } }
In the above code, two coroutines are launched independently to execute API calls for genres and movie titles. This allows the calls to be made concurrently, maximizing performance by reducing waiting time. The results are then posted to their respective LiveData objects for further processing.
Conclusion:
Handling multiple API calls in Android applications requires careful consideration of dependencies, concurrency, and performance. By utilizing Kotlin coroutines and appropriate await functions, we can effectively manage sequential and concurrent API calls.
When API calls have dependencies, sequential execution using async and await ensures proper order. For concurrent execution with result processing, awaitAll simplifies handling multiple results. And when independent API calls are needed, launching coroutines independently optimizes performance.
By following these best practices, developers can build robust and efficient Android applications that effectively handle multiple API calls, providing users with a seamless experience.
Remember to adapt the provided code snippets to your specific project requirements and consider additional error handling, caching mechanisms, and best practices to ensure the reliability and performance of your application.
CodeBase: Example Code base for handling Multiple APIs
Happy coding!
Author:
Shridhar Choudhari
Software Developer. Cumulations Technology
Cumulations Technologies is a mobile app development company in bangalore. Specialized in Android app development and iOS app development