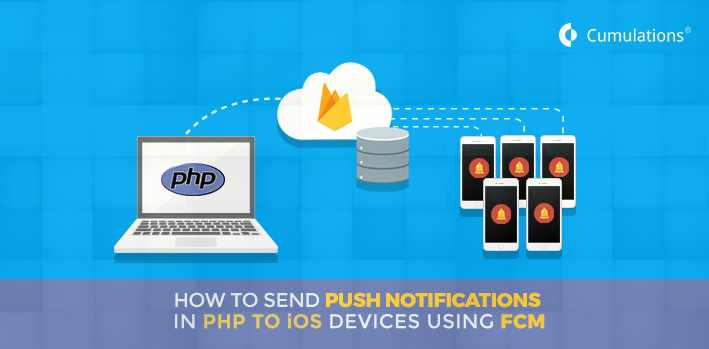
For sending push notifications to iOS devices, we are going to use FCM (Firebase Cloud Messaging) service.
FCM is a new version of GCM (Google Cloud Messaging) service. It is a cross-platform messaging service, used to send notifications that are displayed to the user. We can use any of three ways to send messages to the client app – single devices, groups of devices or devices subscribed to topics. It also sends acknowledgments back to the server. We can use FCM for iOS clients, Android clients, Unity clients, web clients and more. FCM is reliable and battery-efficient.
Here are some steps to follow.
1) Go to the Firebase console https://console.firebase.google.com. (Login using Google account)
2) Create a new project
3) Click on “Add Firebase to your iOS app” button and enter iOS bundle ID (example: com.yourapp.ios) then click on “REGISTER APP”
4) Download configuration file “GoogleService-Info.plist” and move it to the app project folder
5) To configure FCM to APNs (Apple Push Notification service), we need APNs certificate. To generate APNs certificate go to Apple developer console and generate a certificate in .p12 extension
6) In Firebase console, go to “Project settings” then click on “CLOUD MESSAGING”. Upload the APNs certificate (.p12 file) in iOS app configuration
7) To check if the FCM is successfully configured with APNs, send first push notification from Firebase notification console
8) To send push notifications using PHP script, use PHP script written below. Replace with iOS device FCM token and replace with Firebase server key
Note: APNs certificate (.p12 file) has an expired date, so it should be always up-to-date and Firebase may upgrade the server key. You can use Legacy server key but Firebase recommends to use newest server key.
$url = "https://fcm.googleapis.com/fcm/send";
$token = "";
$serverKey = '';
$title = "Title";
$body = "Body of the message";
$notification = array('title' =>$title , 'text' => $body, 'sound' => 'default', 'badge' => '1');
$arrayToSend = array('to' => $token, 'notification' => $notification,'priority'=>'high');
$json = json_encode($arrayToSend);
$headers = array();
$headers[] = 'Content-Type: application/json';
$headers[] = 'Authorization: key='. $serverKey;
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST,
"POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, $json);
curl_setopt($ch, CURLOPT_HTTPHEADER,$headers);
//Send the request
$response = curl_exec($ch);
//Close request
if ($response === FALSE) {
die('FCM Send Error: ' . curl_error($ch));
}
curl_close($ch);