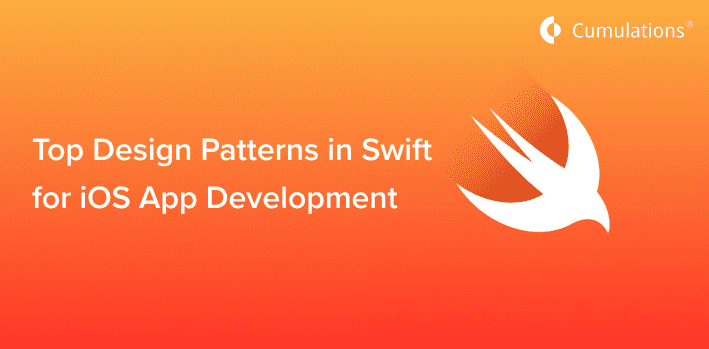
Swift is Apple’s own coding language that was launched in 2014. The immensely popular and powerful tool helps to develop a range of versatile iOS mobile apps for various operating systems.
One of the most significant aspects of using Swift is finalizing the design patterns to be used as well as the right ways to implement them. Because Swift is a relatively new language, a lot of developers struggle with the right choice. Being able to use the accurate design pattern is a prerequisite to developing high-quality, functional, and stable applications.
In this article, we will take a look at the most commonly used design patterns in Swift to help you to make a smarter choice.
What are Design Patterns?
In simple words, a design pattern is a solution to a specific problem encountered while designing the app’s architecture. However, it is important to understand that these design patterns are not any piece of code that can be simply pasted into the application. Instead, they are a concept that demonstrates how a problem is solved. It is like a template that explains how to write the code and solve the problem.
Types of Design Patterns
Now that you have understood what a design pattern is, it is also important to know about the common types. Here are the three types of software design patterns in Swift.
Creational: Creational design patterns are related to object creation mechanisms. They help to offer accurate evidence to represent objects so that can be used in that specific situation. The most common creational design pattern methods are Factory, Singleton, Builder, Abstract, and Prototype.
Structural: These design patterns help to simplify the design by identifying the easy way of instantiating relationships between objects and classes. Commonly used structural patterns include Adapter, Façade, Bridge, Composite, and Proxy.
Behavioral: Behavioral design patterns show common communication patterns that exist between entities. Chain of Responsibility, Command, Template Method, and Iterator are some of the popular behavioral design patterns.
Now let us look at the top 5 design patterns in Swift that are most frequently used for developing iOS apps.
Top Design Patterns in Swift
1. Builder
This is a creational design pattern that allows the developers to build complicated objects from simple ones using a step by step methodology. The design pattern can be used to create one code for various object views.
This is used when you need to compose highly complex objects. Typically, the initialization of code for such complex objects is concealed within a constructor that includes multiple parameters. Now by using the Builder design pattern, you can separate the construction of such an object from its own class. This not only simplifies the code but also breaks the object development into multiple easy steps.
When to use:
When you need to create complicated objects
When you require different views of a particular object
When you want to avoid using telescopic constructor due to its complexity of code
2. Adapter
This is a structural design pattern that facilitates two or more objects with an incompatible interface to seamlessly work together. The pattern essentially transforms the interface of the object so it can easily adapt itself to a different object.
Must read: Apple iPhone 12 Launch Event Highlights
What this design pattern does is wrap the object completely, concealing it from another object. Thus, it helps in connecting different objects to be used together within the app.
When to use:
When you want to add a third-party object in your app but its interface doesn’t match with the rest of the code
When you are using several subclasses in one app or cannot extend the superclass
3. Decorator
Decorator is a structural design pattern that helps to extend the functionalities of the existing objects by wrapping them in useful wrappers. Owing to this, the design pattern is popularly called the Wrapper design pattern.
To use this, the target object is wrapped inside another wrapper object that exhibits newer functionalities. The process triggers the basic behavior of the target object along with adding its own behavior to the result.
The Decorator pattern can also be used to wrap more than two objects together. The only criterion is that the objects should possess the same interface.
When to use:
When it is not possible to add new functionalities to the object through inheritance
When you want to add functionalities to the objects, but at the same time, hide them from the code
4. Façade
This is another structural design pattern that offers a simple interface to a framework, a library, or a set of classes. Using this, you simply don’t need to show several methods with different interfaces.
Façade helps to create your own class and wrap the other objects in it to simplify the code and interface for the users. The simplified interface offers only those features that a client needs while hiding all the others. It is also very helpful when it comes to decomposing the subsystems into multiple different layers.
When to use:
When you want to offer a unified and straightforward interface to a complicated subsystem
When you need to decompose a subsystem into different layers
5. Template Method
This is a behavioral design pattern that is used to outline a skeleton for the algorithm as well as delegate responsibility to the subclasses. It offers flexibility to the subclasses to reevaluate certain steps of the algorithm without imposing any change in the overall structure.
The algorithm is categorized into multiple steps and each of these steps is explained in different methods using the template method.
When to use
When you require subclasses to extend a simple algorithm within altering the structure
When you have multiple classes responsible for similar actions
Apart from these five, there are few other design patterns in Swift that are readily used by iOS developers around the world.
1. MVP
A lot of you must have heard of MVC, the most common iOS architecture design pattern. MVC was also adopted as the official design pattern by Apple. However, it had a few glitches, including lack of distribution of application components and low test coverage. To improve the situation, MVP came into the picture, adding the main component which is the Presenter. This was engineered to enable automated unit testing as well as enhance the bifurcation of concerns in presentation logic.
In greater detail, MVP comprises three components:
Model: This is an interface responsible for domain data, i.e. data that has to be presented or else acted upon in the GUI.
View: View is responsible for the presentation layer.
Presenter: The newest component, this acts as the middle man between the above two. It responds to the user’s actions performed on the View, extracts data from the Model, and formats it for display in the View.
2. MVVM
MVVM stands for Model-View-ViewModel. It is a robust UI design pattern that separates the UI code from the business and presentation logic of an application. As per MVVM, each application is categorized into three different components, which are as follows:
The Model: This is an absolutely independent part that can be used across several applications. It defines core types and executes application business logic.
The View: This component defines the appearance, layout, and structure of the UI. It responds to the user’s actions and informs the ViewModel about the same.
The ViewModel: This part is responsible for wrapping the model and offering state to the UI components. It also outlines actions that can be used by the View to pass events to the model.
3. Viper
This is another commonly used architectural pattern like the MVC and MVVM. However, in this, the code is further separated by single responsibility.
Every single letter in Viper corresponds to an architectural component: View, Interactor, Presenter, Entity, and Router.
View: This part comprises the code to display the app interface to the users and get their feedback. It then alerts the Presenter about the user’s response.
Interactor: This component mediates between the data and the presenter.
Presenter: This is the most important component, often referred to as the “traffic cop” of the architecture. It is the only class to communicate with all other components. The presenter directs the data between the view and interactor, calls the router for wire-framing, and calls the view to update the UI.
Entity: This represents the application data.
Router: This component manages the navigation between screens.
Wrapping Up
This was our roundup of the top 5 design patterns in Swift for app development. Picking the right design pattern is crucial to developing an app that is fully-functional and secure. These design patterns also make the app easy to maintain and upgrade. Thus, using them in your app will not just help you to simplify development but also ensure superior code quality.
Other read: How to use Alexa on the Apple Watch